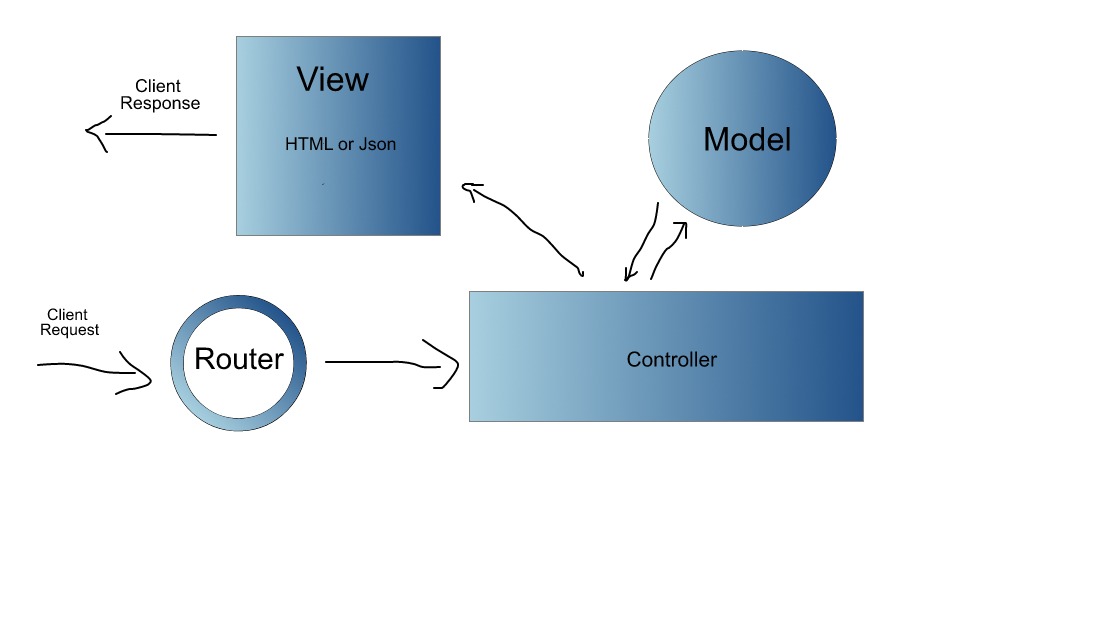
MVC is a framework for building applications
URL | Controller application/controllers/ |
Action | Template application/templates/ |
Parameters |
---|---|---|---|---|
http://localhost/users/register | usersController.js | register() | users/register.html | null |
http://localhost/users/register/acc_type | usersController.js | register() | users/register.html | ['acctype'] |
http://localhost/users/register/acc_type/param2 | usersController.js | register() | users/register.html | ['acctype','param2'] |
http://localhost/users/login | usersController.js | login() | users/login.html | null |
//file application/controllers/usersController.js
module.exports ={
usersController:class {
register(){ //Action here
}
}
}
We can add multiple custom routes with different names, for example we can map http://localhost/members/signin
to usersController and login() method. To do this need to add particulars in application/config/router.js , which has been shown in following lines.
module.exports ={
ROUTES: [
{
path:'users/login/',
// ---- for dynamic parameters ----
// path:'users/login/**',
redirect : {
controller:'users', action:'signin', param:[]
}
}
// use comma and add more
]};
Note :-Calling Router parameters like ControllerName, Action, Params and Base URL inside the Controller Class.
module.exports ={
MEAGER:{
scope:{ }
},
usersController:class {
constructor(MEAGER){
this.routes = MEAGER.routes;
}
}
}
* Need to change DFAULT->encryption->key = "azAZ09" a-z A-Z 0-9
constructor(MEAGER){
this.utilities = this.MEAGER.utilities;
}
let encrypted = this.utilities.encrypt('hello 123');
let decrypted = this.utilities.decrypt(encrypted);
this.postData.then(res =>{ console.log(res); });
module.exports ={
MEAGER:{
scope:{
mongo:true
}
},
usersController:class {
constructor(MEAGER){
this.mongo = MEAGER.imports.mongo;
}
}
}
// To Fetch without filters this.mongo.find({ },'users') .then(dbData=>{ // dbData will contain json records }) .catch(error=>{ console.log('Error !',error); });
// To Fetch with filters * this.mongo.find({ name:'arun', password:'123', },'users') .then(res=>{ // dbData will contain json records }) .catch(error=>{ console.log('Error !',error); });
this.mongo.insert({
name:'arun',
password:'123',
mobile:'87867676577'
},'users')
.then(res=>{
if(res=='success'){
}
})
.catch(error=>{
console.log('Error !',error);
});
this.mongo.update({
name:'arun',
},{
name : 'new name here'
},'users')
.then(res=>{
if(res=='success'){
}
})
.catch(error=>{
console.log('Error !',error);
});
this.mongo.remove({
name:'arun',
},'users')
.then(res=>{
if(res=='success'){
}
})
.catch(error=>{
console.log('Error !',error);
});
this.mongo.count({
name:'arun',
},'users')
.then(res=>{
// res will have count of records
})
.catch(error=>{
console.log('Error !',error);
});
npm install meagerjs
meagerjs_clone
cd meagerjs
node start